C Functions
C structures, c reference, c operators.
Operators are used to perform operations on variables and values.
In the example below, we use the + operator to add together two values:
Although the + operator is often used to add together two values, like in the example above, it can also be used to add together a variable and a value, or a variable and another variable:
C divides the operators into the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators

Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations.
Assignment Operators
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Advertisement
Comparison Operators
Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions.
The return value of a comparison is either 1 or 0 , which means true ( 1 ) or false ( 0 ). These values are known as Boolean values , and you will learn more about them in the Booleans and If..Else chapter.
Comparison operators are used to compare two values.
Note: The return value of a comparison is either true ( 1 ) or false ( 0 ).
In the following example, we use the greater than operator ( > ) to find out if 5 is greater than 3:
A list of all comparison operators:
Logical Operators
You can also test for true or false values with logical operators.
Logical operators are used to determine the logic between variables or values, by combining multiple conditions:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

In-Depth Guide to the Division Assignment Operator (/=) in C Programming
The division assignment operator (/=) is an incredibly useful operator in C that allows you to divide a variable by another value and assign the result back to the original variable in one concise statement. However, there are some intricacies to using /= properly and effectively that are important to understand.
In this comprehensive guide, we will cover everything you need to know about the /= operator, including:
- What exactly /= does
- The syntax and usage of /=
- How /= works under the hood
- Common errors and pitfalls with /=
- Best practices for using /=
- Real-world examples and advanced use cases for /=
- Comparisons to similar operators in other languages
- Compiler optimization of /=
Whether you‘re a beginner looking to solidify your foundational C knowledge or a seasoned developer looking to deepen your understanding, this guide has something for you. So let‘s dive in!
What Exactly Does the /= Operator Do?
At its core, the /= operator divides the variable on the left-hand side by the expression on the right-hand side. The end result is then assigned back to the variable on the left.
For example:
Here, x starts with an initial value of 10. The /= operator then divides x by 5, which results in 2. This 2 is assigned back to x, so x now contains the value 2 after this statement executes.
In essence, x /= y; is equivalent to writing:
Except that /= lets you accomplish this division and assignment in one compact operation rather than separating it into two steps. This makes your code cleaner and more efficient.
Integer vs Floating Point Division
It‘s worth noting that /= handles division differently depending on the data types involved:
- With integers, /= performs truncating division, discarding any fractional remainder
- With floats/doubles, /= preserves the fractional remainder
float y = 7.0 / 2.0; // y = 3.5
This distinction affects how you apply /= in practice. Use integer /= for fast whole number math. Use float/double /= when you need precision.
The Syntax and Usage of /=
The syntax for the /= operator is straightforward:
- variable is any valid variable name
- expression is any valid expression that resolves to a value
float balance = 100.50; balance /= 1.05; // Divide balance by 1.05
The variable and expression can be of any data type, as long as it makes sense for them to be divided. Typically, /= is used with integer, float or double types.
It‘s also worth noting that the variable and expression don‘t have to be the same type. C will automatically convert between numeric types with a few reasonable exceptions.
Here C converts the integer count to a double so it can properly divide by 2.0.
How /= Works Under the Hood
When you use /=, C handles the division and assignment in a specific order:
- The expression on the right side is evaluated to produce a value
- This value is used to divide the current value of the variable on the left side
- The result of the division is implicitly converted to match the type of the variable on the left
- This converted result is assigned back to the variable on the left
Looking at an example will help illustrate:
Here‘s what happens step-by-step:
- y contains 2.5 already so no need to evaluate
- 2.5 is used to divide 10, which results in 4
- This 4 is converted from a float to an int, truncating the value to just 4
- This final 4 is assigned back to x
After this statement, x will contain 4.
As you can see, C handles some subtle type conversion and clamping whenever types are mismatched. This helps make /= reasonably robust without requiring perfect matches.
Compiler Optimizations
When you use /=, compilers like GCC and Clang can optimize the resulting machine code to be as fast as possible using tricks like:
- Strength reduction to replace divisions with faster multiply operations
- Loop invariant code motion of constants outside of loops
- Integer division shortcuts when the divisor is a power of 2
So while /= may seem simple from a C code perspective, rest assured that it maps down efficiently to backend code.
Common Errors and Pitfalls with /=
Although /= is designed to be easy-to-use, there are some common mistakes programmers make. Being aware of these will help you avoid shooting yourself in the foot.
1. Dividing by Zero
In mathematics, dividing by zero is undefined. Unfortunately, C does not handle divide by zero elegantly. If the expression in a /= operation equates to 0, your program will crash immediately with a divide by zero error.
x /= y; // CRASH! Divide by zero error
To prevent this, always check that variables are non-zero before using /=:
if (y != 0) { x /= y; }
2. Operator Precedence Mix Ups
The /= operator has relatively high precedence in C, just below other arithmetic and bitwise operators. This can lead to unintended results in complex expressions:
int y = 10; y /= 2 + 3; // y = 2, not 1!
Use parenthesis liberally to clarify intended order of operations:
int y = 10; y /= (2 + 3); // y now 1
Best Practices for Using /=
Once you understand the basics of /=, using it effectively really comes down to following best practices:
- Use /= for integer math when you need both the quotient and remainder
- Use /= for float/double math when you need full precision
- Avoid hard-coding literal values with /=, use named constants instead
- Always check for divide by zero before using /= on variables
- Use parenthesis to explicitly indicate order of operations
- Minimize divides in tight loops for better performance
- Consider creating reusable divide functions rather than littering /= throughout code
Adhering to these simple guidelines will help you avoid surprises and write robust code that does exactly what you intend it to do.
And as with any construct in C, carefully document instances of /= so your thought process is clear to others reading your code. Well commented /= statements are invaluable down the road.
Real-World Examples and Advanced Use Cases
Now that we‘ve explored the fundamentals of /=, let‘s look at some advanced real-world examples that demonstrate when to reach for it:
Computer Graphics and Physics Engines
In computer graphics and physics simulations, /= is used heavily in vector math:
void normalize(Vector v) { float mag = sqrt(v->x v->x + v->y v->y + v->z v->z); v->x /= mag; v->y /= mag; v->z /= mag; }
Here /= divides each component by the total vector magnitude to produce a normalized direction. This feeds into lighting, mechanics, and more.
Numerical Analysis Algorithms
In numerical methods, /= appears in core algorithms like gradient descent:
float learningRate = 0.01;
// Calculate derivative float xDerivative = numericalDerivative(x);
// Gradient descent update x -= xDerivative * learningRate;
Which is just a wrapped usage of /= on x to take a step downhill. As you can see, /= works great with numerical computing.
Exponential Decay Models
In physics and engineering models, /= can implement exponential decay:
float remaining = initial;
while (remaining > 0.01) {
By dividing the value each iteration by a scaled exponent, /= produces the classic downward curve.
Key Takeaways
The /= operator is one of those deceptively simple but extremely useful constructs in C. By combining division and assignment in one step, it streamlines code and provides a compact way to reduce values.
However, as we explored, there are some pitfalls to be aware of like precedence and divide by zeros. Used carefully while following best practices, /= can be an indispensable tool in your C programming toolbox.
Understanding exactly how it works under the hood and when best to utilize it will pay dividends in writing stable C programs that effectively harness the power of /=. Hopefully this guide gave you ideas for how to specifically apply it and level up your C skills.
- Kernighan, Brian W., and Dennis M. Ritchie. 1988. The C Programming Language. Prentice Hall. Accessed March 9, 2023. https://en.cppreference.com/w/c
- Griffiths, David, and Dawn Griffiths. 2022. Head First C: A Learner‘s Guide to Real-World Programming with C and GNU Development Tools. O‘Reilly Media. Accessed March 9, 2023.
- Wikipedia contributors. "Operators in C and C++." Wikipedia, The Free Encyclopedia. Accessed March 9, 2023. https://en.wikipedia.org/wiki/Operators_in_C_and_C%2B%2B
Related posts:
- Mastering the Art of foreach Loops in C
- Demystifying Integer Division in C
- Mastering Sigaction()-Based Signal Handling in C
- Extracting C Source Code from .SO Shared Object Files
- The Definitive Guide to Pipe System Calls in C
- Comparing Two Strings and Returning the Larger One in C
- A Deep Dive into the sem_open() Function in C
- Mastering the Arrow: An Expert‘s Guide to C‘s -> Operator
Similar Posts
A comprehensive guide to using xfs_repair.
As a full-time Linux filesystem architect and lead XFS developer for over 15 years, I have…
How to Contact Discord Support: An Expert Troubleshooting Guide
As a full-stack developer and habitual Discord power user, I‘ve encountered about every tech issue you…
How to List Installed Packages on Ubuntu: A Comprehensive Guide
Knowing the installed packages on your Ubuntu system is crucial for several reasons. You may need…
Demystifying Docker and Portainer: A Developer‘s Perspective
Microservices and containerization are revolutionizing application development and deployment. As per the 2022 Stack Overflow survey,…
What is the Purpose of WORKDIR in Dockerfile?
A Dockerfile is a text file that contains a set of instructions to build a Docker…
Expert Guide: Installing and Hardening Docker on Kali Linux
Container technology adoption has accelerated enormously in recent years. According to [Red Hat][red1], usage of containers…
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.


Assignment Operators In C | A Complete Guide With Detailed Examples
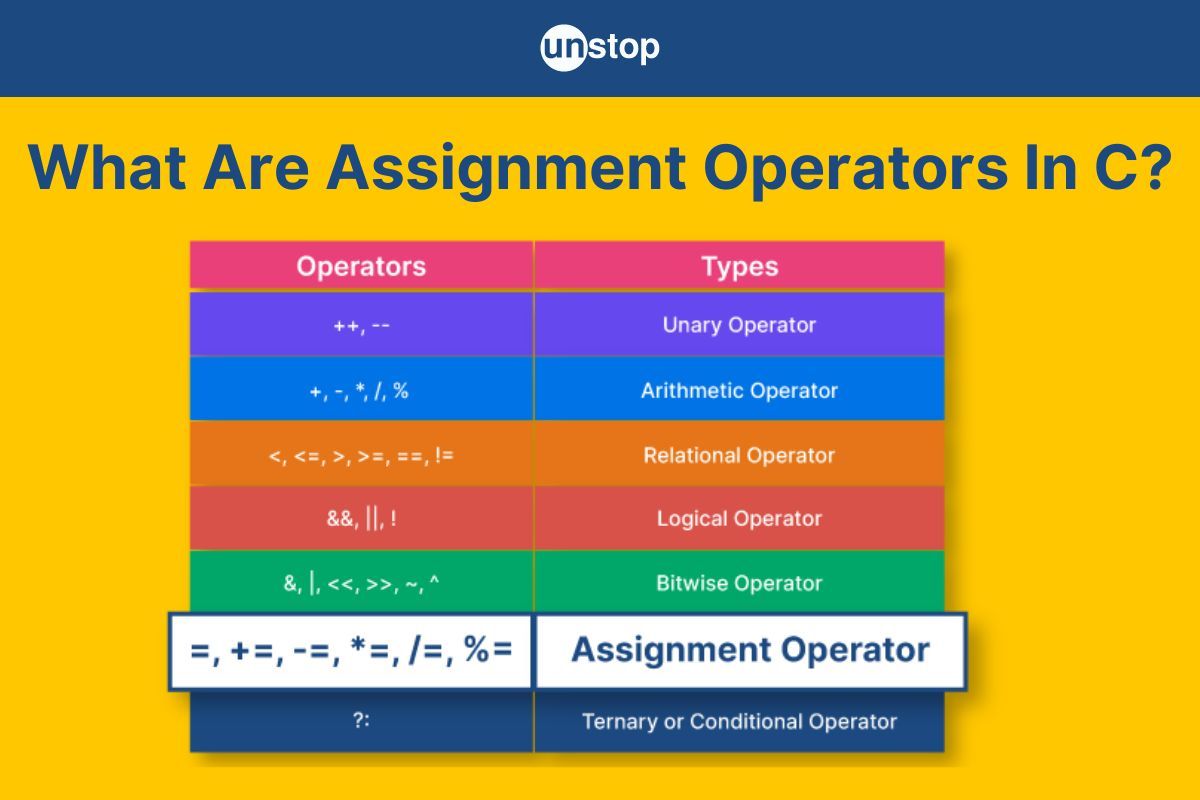
Table of content:
What are assignment operators in c, how assignment operators in c work step-by-step explanation, types of assignment operators in c, bitwise assignment operators in c.
- Precedence & Associativity Of Assignment Operators In C
Table Of Assignment Operators In C
Relational vs. assignment operators in c, frequently asked questions.
Operators are symbols or special characters that perform specific operations on one or more operands. They enable various computations and manipulation of data/ operands in C programs. These operands can be variables, constants, or expressions. It is important to understand the concept of various types of operators to utilize them effectively in writing efficient C code. In this article, we will focus on assignment operators in C.
Other types of operators include arithmetic, bitwise, logical, relational, ternary/ conditional, etc. Collectively, they enable complex computations, comparisons, and control flow within programs, allowing developers to manipulate data in a variety of ways.
In simple terms, assignment operators in C programming are used to assign values to variables. This includes assigning initial value as well as updating the value of a variable with a new value based on a certain operation or calculation. The most commonly used assignment operator is the equal-to operator (=), which assigns the right-hand side value to the variable on the left-hand side of the operator.
For example, the expression x = 5 assigns the value of 5 to the variable x.
Other assignment operators in C include addition assignment (+=), subtraction assignment (-=), multiplication assignment (*=), division assignment (/=), and modulus/ modulo assignment (%=). They are also referred to as compound assignment operators. As their name suggests, they are used to assign the resultant value of addition, subtraction, multiplication, division, and modulus operations (respectively) to the left-side variable/ operand.
For example, the equation x += 5 is equivalent to x = x + 5. It updates the value of x by adding 5 to its current value.
These assignment operators can be combined with other operators to perform more complex operations in a single statement. For example, x += y * 3 updates the value of x by adding three times the value of y to its current value.
Using basic assignment operators can help make code more concise and easier to read and improve performance by reducing the number of operations required. However, it is important to use them carefully and avoid unintended consequences, such as side effects or errors. We will discuss each of these assignment operators in detail ahead, but first let's look at the basic syntax for this.
Syntax For Assignment Operators In C:
variable_name operator expression;
Here,
- The variable_name refers to the name/ identifier of the variable to whom you are assigning (or updating) a value.
- The term operator refers to the respective assignment operator you are using.
- Expression is the right-hand operand, i.e., it refers to the value you assign to the variable.
Here are some examples of assignment operators and their syntax:
x = 5; // assigns the value of 5 to the variable x y += 10; // adds 10 to the current value of y and updates y with the result z *= 3; // multiplies the current value of z by 3 and updates z with the result
Given below is a stepwise description of the working mechanism of assignment operators in C.
- Step 1 (The variable): The first step is to select the variable that we want to assign a value to. This variable can be of any data type, such as integer, float, or char .
- Step 2 (The operator): The second step is to select the assignment operator that we want to use. As mentioned, the most commonly used assignment operator is the simple assignment operator (=), to assign the value of the right side operand to the left side operand.
- Step 3 (The value or expression): The third step is to provide the value (or expression for calculating value) we want to assign to the variable. This value can be a constant or an expression that combines variables and constants using arithmetic or logical operators .
- Step 4 (The evaluation): After that, the compiler evaluates the expression on the right-hand side of the operator. This evaluation involves performing any necessary logical or arithmetic operations based on the precedence rules of the operators.
- Step 5 (The assignment): Finally, it assigns the evaluated value to the variable on the left-hand side of the assignment operator. If we are updating the value, this assignment replaces the previous value of the variable with the new value.
Let's look at a basic C program example illustrating both these approaches of using assignment operators.
Code Example:
The initial values of x and y are: 5, 10 The updated value of x is: 15
Explanation:
We begin the simple C program example by including the <stdio.h> header file for input/output operations.
- We then initiate the main() function , which is the entry point of the program's execution.
- Inside main(), we declare two integer variables , x and y, and assign the values of 5 to x and 10 to y. Then, we print these initial values to the console using the printf() function .
- Next, we use the addition assignment operator (as mentioned in the code comments ) to add the initial value of y to the initial value of x and assign the result to x.
- The operation involves adding the value of y, which is 5, to the value of x, which is 10. The output is, hence, 15.
- Once again, we use the printf() function to display the updated value of x.
All the assignment operators in C programming language can be classified into two major types with subtypes. These types and the operators included in them are mentioned below-
Simple Assignment Operator In C (=)
There is only one assignment operator that falls into this category. This is the simplest assignment operator whose only purpose is to assign the value given on the right to the variable on the left. The basic assignment operator symbol is a single equals sign (=). It is the most commonly used assignment operator in C language. Below is an example of how to use this operator in simple assignment expressions.
The value of x is 5
In the simple C code example , we include the standard input-output header file, <stdio.h>, which contains the library functions for input and output operations in C programming.
- Inside main() funtion , we declare an integer variable named x and initialize it with the value 5 using the simple assignment operator .
- This step reserves a memory location to store an integer value and assigns the value 5 to x.
- Next, we use the printf() function to display the output with a descriptive string message (using formatted string) .
- Here, we use the %d format specifier as a placeholder for the integer value and the newline escape sequence (\n) to shift the cursor to the next line afterwards.
- Lastly, the program successfully completes execution with the return 0 statement . It indicates the successful termination of the main() function and the program as a whole.
It will be easier to understand why we call this operator simple when you check out the other category, i.e., compound operators.
Compound Assignment Operators In C
The compound assignment operators are called as such because they combine a binary operation with the assignment operation. They perform a binary operation on the values of the variable and the value on the right-hand side and then assign the result to the variable on the left-hand side/ left operand.
There are five types of compound assignment operator in C:
- Subtraction Assignment Operator (-=)
- Addition Assignment Operator (+=)
- Multiplication Assignment Operator (=)
- Division Assignment Operator (/=)
- Modulus Assignment Operator (%=)
The advantage of using these operators is that they provide a shorthand notation for performing common operations such as addition, multiplication, division, etc.
Now that you have a broad idea of what compound assignment operators in C are, let's explore each type of assignment operator in detail.
Addition Assignment Operator In C (+=)
The addition assignment operator adds the value given on its right side to the current value of the variable on the left. It then assigns the resultant value back to the variable on the left.
In other words, it adds the initial value of the first variable to the initial value of a second variable and assigns it back to the first variable. Below is a C program example illustrating the use of this compound assignment operator.
The value of x is 8
In the C code example, we first include the essential header files.
- Inside the main() function , we declare an integer variable, x , and initialize it with the value 5 using the simple assignment operator.
- Next, we use the addition assignment operator (+=) to add a value 3 to x (variable on the left side) and assign the result back to it.
- Here, we add 3 to x's initial value and reassign it back. That is, x += 3 is equivalent to x = x + 3, which adds 3 to x's value and stores the result back into x.
- Then, we use the printf() function to display the output to the console, after which the program terminates with the return 0 statement.
Check out this amazing course to become the best version of the C programmer you can be.
Subtraction Assignment Operator In C (-=)
The subtraction assignment operator is used to subtract a given value, mentioned on the right side of the operator, from the initial value of the variable on the left (i.e., the left operand). It then assigns the result back to the original variable on the left. Alternatively, we can also subtract the value of a second variable from the initial variable.
The value of x is 7
In the example C program -
- Inside the main() function , we declare an integer variable x and assign the value 10 to it using the simple operator.
- We then use the subtraction assignment operator (-=) to subtract a value 3 from the initial value of x. The result is assigned back to x using this operator.
- Here, the expression x -= 3 is equivalent to x = x - 3, which means we subtract 3 from 5 (i.e., the value of x), and the value of x is updated with the result.
- After that, we use the printf() function to display the updated value of the variable x to the console.
Multiplication Assignment Operator In C (*=)
The multiplication assignment operator in C (*=) first multiplies a given value (on the right) with the initial/ current value of the variable on the left (left operand). Then, it assigns the result back to the variable on the left. Alternatively, it can also be used to multiply the initial value of a variable by the value of another variable in the program.
The value of x is 20
In the example C code -
- Inside the main() function , we declare an integer variable named x and initialize it with the value 5 using the simple assignment operator.
- Next, we use the multiplication assignment operator to multiply the initial value of x by 4 and then assign the result back to variable x.
- That is, the expression x *= 4 implies x = x * 4, which multiplies the value of x by 4 and stores the result back into x.
- Using the printf() function , we display the updated value of x and a string literal message.
Division Assignment Operator In C (/=)
The division assignment operator (/=) first divides the current/ initial value of the variable mentioned on its left side by the value given on its right. Then, the revised value/ result is assigned back to the variable on the left. Alternatively, we can also use this operator to divide the value of one variable by another variable and then update the value of one.
Let's take a look at an example that illustrates the use of this compound assignment operator in C code.
In the sample C program -
- We declare an integer variable x inside the main() function and initialize it with the value 10.
- Next, we use the division assignment operator (/=) to divide the value of x by 2 and assign the result of this operation back to variable x.
- Here, the expression x /= 2 can be expanded as x = x / 2, which ultimately divides the value of x by 2 and stores the result back into x.
- Finally, we display this output using the printf() function with a formatted string message.
Modulo Assignment Operator In C (%=)
The modulo assignment operator (%=) is a combination of the modulo arithmetic operator and assignment operator. It divides the value of the left-side operand by the right-side operand to calculate the remainder of this division. Then, it assigns the resulting remainder back to the variable on the left. Note that the value on the right can also be the value of another variable in the program.
Look at the example below to better understand how to apply this compound assignment operator in C programs.
The value of x is 3
In the sample C code -
- In the main() function , we declare a variable, x, of integer data type and initialize it with the value of 14.
- Then, we use the modulo assignment operator ( %=) to calculate the remainder from the division of the variable on the left side by a specified value and assign the result back to that variable.
- In this example, we divide the value of x by 4 and reassign the remainder to it.
- That is, x %= 4 is equivalent to x = x % 4, which ultimately calculates the remainder when x is divided by 4 and stores the result back into x.
- This reassigned/ updated value of x is displayed on the console using the printf() function with a formatted string and %d format specifier.
We have already discussed the most commonly used assignment operators in C programing language. There are a few other compound assignment operators that you must know about. These are the bitwise assignment operators, which first conduct the respective bitwise operation on the operands and then assign the result back to the left operand in the expression.
There are three types of bitwise assignment operators in C, which we have discussed ahead.
Bitwise AND Assignment Operator In C (&=)
The bitwise AND assignment operator (&=) performs a bitwise AND operation between the current value of the variable on the left (i.e., the left operand) and the value on the right (i.e., the right operand) and then assigns the result to the variable on the left. The value on the right can also be another variable.
The assignment operator example below showcases how this compound operator works.
The value of x is 4
In the C program sample , we define the main() function (which serves as the entry point) after including the <stdio.h> file.
- We then declare an integer variable called x and use the basic assignment operator to initialize it with the value 12.
- Next, we use the bitwise AND assignment operator (&=) to perform a bitwise AND operation between the value of the variable on the left side and a specified value, and then we assign the result back to that variable.
- The equation x &= 5 , which implies x = x & 5, performs a bitwise AND between the value of x and 5 and stores the result back into x.
- We display the result of this operation to the console with the printf() function , a formatted string, and the %d specifier.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Bitwise OR Assignment Operator In C (|=)
The bitwise OR assignment operator (|=) performs a bitwise OR operation between the current value of the variable on the left and the value on the right and then assigns the result to the variable on the left. The example below showcases the use this compound assingment operator in C.
Output Window :
The value of x is 13
Code Explanation:
In the C code sample -
- In the main() function , we declare an integer variable x and initialize it with the value 12. This reserves a memory location to store an integer value.
- Next, using the bitwise OR assignment operator , we perform a bitwise OR operation between the value of the variable on the left side (i.e., 12) and a specified value (i.e., 5) and assign the result back to the left-side variable, i.e., x.
- The expression x |= 5 leads to the evaluation of x = x | 5, which performs a bitwise OR between the value of x and 5 and stores the result back into x.
- Then, we use the printf() function to display the result/ updated value of left operand x to the console. We also use a formatted string and the %d specifier inside printf().
Left Shift Assignment Operator In C (<<=)
The bitwise left shift assignment operator (<<=) first shifts the bits of the current value of the variable on the left (i.e., left-hand operand) by the number of positions specified by the value on the right. Then, it assigns the result of this operation back to the variable on the left.
The value of x is 40
In this assignment operator example, we first include the essential header file and then initiate the main() function .
- Inside main(), we declare an integer variable x and initialize it with the value 10. This step reserves a memory location to store an integer value and assigns the value 10 to x.
- Next, we apply the left shift assignment operator (<<=) on this variable to perform a left shift operation on its value by a specified number of bits (here, 2) and assign the result back to that variable.
- The equation x <<= 2 expands to x = x << 2, which performs a left shift on the value of x by 2 bits and stores the result back into x.
- We print the new value of the left-hand side variable x to the console using the printf() function .
Right Shift Assignment Operator In C (>>=)
The bitwise right shift assignment operator (>>=) begins by shifting the bits of the initial value of the variable on the left (i.e., left-hand operand) by the number of positions specified by the right-hand value and then assigns the result to the variable on the left.
In the assignment operator C example-
- Inside the main() function (which serves as the entry point for program execution), we declare an integer variable x and initialize it with the value 10.
- Next, we employ the right shift assignment operator ( >>=) to shift the bits of the value on the left side (i.e., 10, the value of x) by a specified number of positions (i.e., 1) to the right. Then, assign the result back to the left-hand side variable.
- The equation x >>= 1 is equivalent to x = x >> 1, which performs a right shift operation on the value of x by 1 position and stores the result back into x.
- We publish this updated value of the variable x to the console using a formatted string and %d specifier inside the printf() function .
Also Read: Shift Operators In C | The Ultimate Guide With Code Examples
Precedence & Associativity Of Assignment Operators In C
In C, operators have a defined precedence that determines the order in which expressions are evaluated when multiple operators are present. Precedence helps in resolving which operator should be applied or evaluated first in an expression. Associativity, on the other hand, defines the direction in which operators of the same precedence level are processed.
Precedence of Assignment Operators In C
All assignment operators in C (=, +=, -=, *=, /=, %=, etc.) have lower precedence compared to most other operators, such as arithmetic operators (+, -, *, /), relational operators (<, >, <=, >=) , and logical operators (&&, ||). This means that in an expression where assignment operators are used alongside other operators, the other operators are evaluated first before the assignment takes place.
In other words, the other operators take precedence over assignment operators when evaluating expressions in C programs. For example:
int a = 5; int b = 10; int c = a + b * 2;
In this expression, the multiplication operator (*) has higher precedence than the addition operator (+), and both have higher precedence than the assignment (=). Therefore, b * 2 is evaluated first, then the result is added to a, and finally, the sum is assigned to c.

Assignment Operator Associativity In C
Assignment operators in C have right-to-left associativity. This means that when multiple assignment operators are used in a single expression, the expression is evaluated from right to left.
int a, b, c; a = b = c = 10;
In this case, the value 10 is first assigned to c, then the value of c is assigned to b, and finally, the value of b is assigned to a. Since every assignment operator associates from right to left, this evaluation happens from right to left.
This associativity of assignment operators in C is particularly important to understand when chaining assignments or combining them with other operations.
Here is a table listing all the assignment operators in C programming with a brief description and example.
Both the relational and the assignment operators serve different purposes and have distinct characteristics in programming languages like C. Assignment operators deal with assigning values to operands/ variables. In contrast, relational operators focus on making comparisons between operands, thus enabling logical decision-making in control flow statements .
Here are some points highlighting the differences between relational and assignment operators in C:
Purpose | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators are used to assign values or expressions to variables. They perform the task of storing a value in a variable.
Relational Operators: Relational operators are used to compare values or expressions. They determine the relationship between two operands and produce a Boolean result (true or false) based on the comparison.
Syntax | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators are typically represented by a single character followed by an equal sign, such as (=), (+=), (-=), (*=), etc.
Relational Operators: Relational operators are represented by two or more characters, including equality operators (==, !=), other relational operators (<, >, <=, >=), etc.
Operation | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators in C perform the task of assigning a value or expression to a variable. They modify the value of the left-hand side variable based on the right-hand side value or expression.
Relational Operators: Relational operators compare the values of two operands and return a Boolean result indicating whether the comparison is true or false.
Result Type | Relational Vs. Assignment Operators In C
Assignment Operators: In case of the simple assignment operator, the result is not a value in itself but the assignment of value to the variable on the left-hand side of the operator. But in case of compound assingment operators in C the result is the value assigned to the variable on the left-hand side of the operator, which can be used in further expressions or assignments.
Relational Operators: The result of a relational operator is a Boolean value, either true or false, indicating the outcome of the comparison.
Usage | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators in C are primarily used for variable assignments, updating variables, and performing calculations involving the current value of a variable.
Relational Operators: Relational operators are used in conditional statements (if, while, for) and expressions that require comparison between variables or values.
Precedence | Relational Vs. Assignment Operators In C
Assignment Operators: Assignment operators in C have lower precedence compared to most other operators, which means they are evaluated after most other operations in an expression.
Relational Operators : Relational operators have higher precedence than assignment operators, allowing them to be evaluated before assignment operations.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here .
The assignment operator in C is one of the most important and commonly used operators in the language. It assigns values to variables, which is essential for writing any program. Compound assignment operators can be used to make code more concise and efficient. These assignment operators are fundamental operators that allow programmers to store and manipulate data effectively throughout their programs. It is important to understand the different types of assignment operators and how to use them correctly to write and run efficient and effective C code .
Also Read: 100+ Top C Interview Questions With Answers (2024)
Q. What is an assignment operator in C?
An assignment operator is an operator in the C language that assigns a value to a variable. This could be a direct value (as in initialization) or the value that results from a compound operation (like the result of addition, subtraction, multiplication, etc. operations).
The most commonly used assignment operator is the equal sign (=), the basic assignment operator. It assigns the value on the right-hand side to the variable on the left-hand side of the operator.
Q. What is the difference between a simple assignment operator and a compound assignment operator?
The simple assignment operator, the equal-to operator (=), assigns a single value to a variable. On the other hand, a compound assignment operator in C (binary operator) performs a binary operation (such as addition, subtraction, multiplication, etc.) between the current value of a variable and another value or expression and then assigns the result to the variable. Its examples are- addition assignment (+=), multiplication assignment (*=), modulo assignment (%=), etc.
Q. What happens if I use an assignment operator with incompatible types in C?
Using an assignment operator with incompatible types in the C programming language results in a compilation error . C is a statically typed language, meaning variables must be explicitly declared with their data types, and the compiler enforces strict type-checking during the compilation process . If an attempt is made to assign a value of one type to a variable of a different, incompatible type without an explicit type conversion , the compiler will raise an error.
For example, trying to assign a character ('A') to an integer variable declared as int will lead to a compilation error. This mechanism helps catch potential type-related errors early in the development process, ensuring that the program adheres to the specified type rules and preventing unexpected behavior during runtime.
Q. Can I chain multiple assignment operators together in C language?
In the C programming language, it is possible to chain multiple assignment operators together in a single statement. That is, you can assign the same value to multiple variables in one line by chaining assignments. For example, the following code is valid in C:
int a, b, c; a = b = c = 5; // This is not allowed in C
This assigns the value 5 to all three variables (a, b, and c). The expression is evaluated from right to left due to the right-associative nature of the assignment operators in C.
Q. Are there any precedence rules for assignment operators?
Yes, there are precedence rules for assignment operators in C language. The assignment operator (=) has a lower precedence than most other operators. This means that when an expression contains both assignment and other operators, the assignment will be performed after the evaluation of the other operators.
For example:
int a, b, c; a = b = c = 5 + 3 * 2;
In this example, the multiplication (*) has higher precedence than the assignment (=). So, 3 * 2 is evaluated first, resulting in 6. Then, 5 + 6 is evaluated, resulting in 11. Finally, the assignment is performed from right to left, so c, b, and a will all be assigned the value 11.
It's important to note that associativity also plays a role. The assignment operators in C are right-associative, which means that when multiple assignments appear in a row, they are evaluated from right to left. In the example above, c = 5 + 3 * 2 is evaluated first, followed by b = c, and finally a = b.
Now that you know all about assignment operators in C, check out some other interesting topics:
- Increment And Decrement Operators In C With Precedence (+Examples)
- Keywords In C Language | Properties, Usage, Examples & Detailed Explanation
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Ternary (Conditional) Operator In C Explained With Code Examples
- For Loop In C | Structure, Working & Variations With Code Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
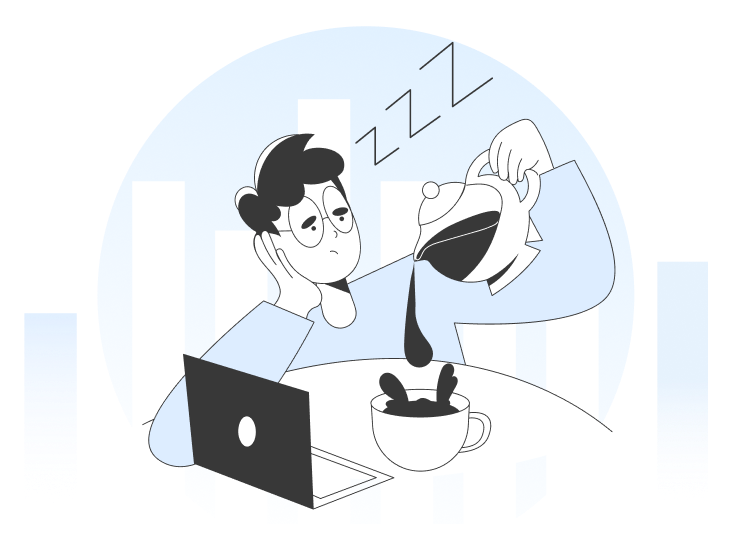
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

to our newsletter
Blogs you need to hog!
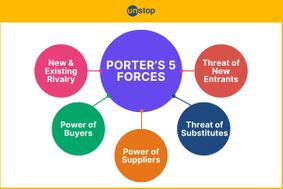
Porter's 5 Forces: Comprehensive Guide To This Analytical Tool
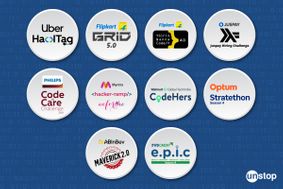
How To Organize Hackathons & Coding Competitions
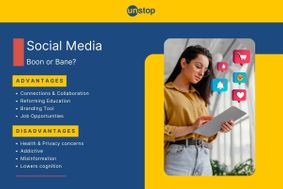
Social Media: Boon Or Bane? Delving Deep Into The Debate
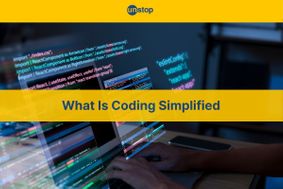
What Is Coding | Role, Working, How To Learn & More Simplified
TutorialKart
- C Programming
- C Datatypes
- C Variables
- C Constants
C Operators
- C Ternary Operator
- C Structures
- C File Operations
C Operators are symbols that take one or two operands, perform a specific action on these operands, and returns the result.
Operators can be classified into following groups.
- C Assignment Operators
- C Arithmetic Operators
- C Logical Operators
- C Relational Operators
- C Bitwise Operators
In this tutorial, we shall go through each of these Operators, and also list out individual tutorials that describe respective operators in detail.
Assignment Operators
Arithmetic operators are used to perform common operations like addition, subtraction, multiplication, division, etc.
The following table specifies symbol, example, and description for each of the Assignment Operator in C programming.
The following are the list of detailed tutorials for Arithmetic Operators.
- C Simple Assignment Operator
- C Addition Assignment Operator
- C Subtraction Assignment Operator
- C Multiplication Assignment Operator
- C Division Assignment Operator
- C Remainder Assignment Operator
- C Bitwise AND Assignment Operator
- C Bitwise OR Assignment Operator
- C Bitwise XOR Assignment Operator
- C Left-shift Assignment Operator
- C Right-shift Assignment Operator
Arithmetic Operators
- C Subtraction
- C Multiplication
- C Increment
- C Decrement
Logical Operators
Logical operators are used to perform boolean operations like AND, OR, and NOT.
The following are the list of detailed tutorials for Logical Operators.
Relational Operators
Relational operators are used to compare given values. These are also called Comparison Operators.
- C Equal To Operator
- C Not Equal Operator
- C Greater Than Operator
- C Less Than Operator
- C Greater Than or Equal To Operator
- C Less Than or Equal To Operator
Bitwise Operators
C Bitwise Operators are used to perform bitwise operations on integer or char operands. Bitwise operations are done at bit level, meaning, operations like AND, OR, XOR, etc., are done between respective bits of the operands.
The following table specifies symbol, example, and description for each of the Bitwise Operator in C programming.
- C Bitwise AND Operator
- C Bitwise OR Operator
- C Bitwise XOR Operator
- C Bitwise Complement Operator
- C Bitwise Left Shift Operator
- C Bitwise Right Shift Operator
In this C Tutorial , we learned about different types of operators in C programming.
Popular Courses
- Salesforce Admin
- Salesforce Developer
- Visualforce
- Informatica
SAP Resources
- Kafka Tutorial
- Spark Tutorial
- Tomcat Tutorial
- Python Tkinter
Programming
- Android Compose
- Kotlin Android
- Bash Script
Web & Server
- Selenium Java
- Definitions
- General Knowledge
01 Career Opportunities
02 beginner, 03 intermediate, 04 advanced, 05 training programs, c programming assignment operators, c programming free course with certificate: learn c in 21 days, what is an assignment operator in c, types of assignment operators in c, 1. simple assignment operator (=), example of simple assignment operator, 2. compound assignment operators, example of augmented arithmetic and assignment operators, example of augmented bitwise and assignment operators, practice problems on assignment operators in c, 1. what will the value of "x" be after the execution of the following code, 2. after executing the following code, what is the value of the number variable, benefits of using assignment operators, best practices and tips for using the assignment operator, about author, live batches schedule view all.

- C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
Code Translation Project
Don't lose in a world of programming languages
C - Division: /
Description.
- par1 - At least one real number
- par2 - At least one real number
- Result - Real numbers Result of the division is always a real number.
- par1 - Any integer
- par2 - Any integer
C Assignment Operators
Answered on: Wednesday 24 January, 2024 / Duration: 20 min read
Programming Language: C , Popularity : 6/10
Solution 1:
Certainly! In C programming, assignment operators are used to assign values to variables. Here are some common assignment operators in C along with examples and outputs:
1. Simple Assignment (=): - The basic assignment operator assigns the value on the right-hand side to the variable on the left-hand side.
2. Addition Assignment (+=): - Adds the value on the right-hand side to the variable on the left-hand side.
3. Subtraction Assignment (-=): - Subtracts the value on the right-hand side from the variable on the left-hand side.
4. Multiplication Assignment (*=): - Multiplies the variable on the left-hand side by the value on the right-hand side.
5. Division Assignment (/=): - Divides the variable on the left-hand side by the value on the right-hand side.
These examples illustrate the usage of various assignment operators in C, demonstrating how they modify the values of variables based on specific operations.
Solution 2:
Assignment Operators in C
Assignment operators are used to assign values to variables. The general form of an assignment operator is:
where variable is the name of the variable to be assigned a value, and expression is the expression whose value is to be assigned to the variable.
There are several different assignment operators in C, each of which performs a different operation. The following table lists the different assignment operators and their descriptions:
| Operator | Description | |---|---| | = | Simple assignment | | += | Addition assignment | | -= | Subtraction assignment | | *= | Multiplication assignment | | /= | Division assignment | | %= | Modulus assignment |
Let's look at some examples of how assignment operators are used in C.
Simple Assignment
The simplest assignment operator is the = operator. This operator simply assigns the value of the expression on the right-hand side to the variable on the left-hand side. For example:
This statement assigns the value of 10 to the variable x .
Addition Assignment
The += operator adds the value of the expression on the right-hand side to the variable on the left-hand side. For example:
This statement adds 5 to the value of x , and then assigns the new value to x . After this statement executes, the value of x will be 15.
Subtraction Assignment
The -= operator subtracts the value of the expression on the right-hand side from the variable on the left-hand side. For example:
This statement subtracts 5 from the value of x , and then assigns the new value to x . After this statement executes, the value of x will be 5.
Multiplication Assignment
The *= operator multiplies the value of the expression on the right-hand side by the variable on the left-hand side. For example:
This statement multiplies the value of x by 5, and then assigns the new value to x . After this statement executes, the value of x will be 50.
Division Assignment
The /= operator divides the value of the variable on the left-hand side by the value of the expression on the right-hand side. For example:
This statement divides the value of x by 5, and then assigns the new value to x . After this statement executes, the value of x will be 2.
Modulus Assignment
The %= operator returns the remainder of dividing the value of the variable on the left-hand side by the value of the expression on the right-hand side. For example:
This statement divides the value of x by 5, and then assigns the remainder to x . After this statement executes, the value of x will be 0.
Operator Precedence
When multiple assignment operators are used in the same expression, the operators are evaluated in the following order:
1. *= , /= , %= 2. += , -= 3. =
For example, the following expression:
is equivalent to the following expression:
Assignment Operators and Side Effects
Assignment operators can have side effects. A side effect is an unintended consequence of an operation. For example, the ++ operator increments the value of a variable by 1. This can have a side effect if the variable is used in another part of the program. For example, the following code:
will assign the value of x to y before incrementing the value of x . This means that y will be assigned the value of 10, and then x will be incremented to 1
Solution 3:
In C programming language, Assignment Operators are used to assign values to variables. There are several types of assignment operators in C, each with its own specific use case. Here are some of the most common assignment operators in C:
1. Simple Assignment Operator (=): This operator is used to assign a value to a variable. For example:
In the above example, the value 10 is initially assigned to variable a . Later, the value 20 is assigned to a , replacing its previous value.
2. Addition Assignment Operator (+=): This operator is used to add a value to a variable and assign the result to the variable. For example:
In the above example, the value of a is increased by 5 using the addition assignment operator.
3. Subtraction Assignment Operator (-=): This operator is used to subtract a value from a variable and assign the result to the variable. For example:
More Articles :
Write a c proogram to find the roots of quadratic equation.
Answered on: Wednesday 24 January, 2024 / Duration: 5-10 min read
Programming Language : C , Popularity : 3/10
time include c
Programming Language : C , Popularity : 9/10
c program to find the factorial of a number
Programming Language : C , Popularity : 8/10
commentaire c
Programming Language : C , Popularity : 10/10
Splash Timer Flutter
Programming Language : C , Popularity : 6/10
how to return two values in c
C style array, c concatenate strings, c programming language.
Programming Language : C , Popularity : 4/10
Counting Sort C
C bit access struct, turn a char into an int in c.
Programming Language : C , Popularity : 5/10
c read file content
How to pass an array of structs as an argument in c.
Programming Language : C , Popularity : 7/10
c how to find size of array
Vim set tab to 4 spaces, markdown c sharp, conda empty environment, parsererror: error tokenizing data. c error: expected 1 fields in line 6, saw 3, clrscr in c, how to change an array in a function in c, making a programming language in c, array value from user c, reattach screen linux, bootsrap textbox, arduino millis, how to run shell command ctrl + c in python script, block a website on mac, floyd's triangle in c.

IMAGES
COMMENTS
Sep 21, 2024 · The simple assignment operator is =. C also supports shorthand assignment operators that combine an operation with assignment, making the code more concise. Key Topics: Simple Assignment Operator; Shorthand Addition Assignment (+=) Shorthand Subtraction Assignment (-=) Shorthand Multiplication Assignment (*=) Shorthand Division Assignment (/=)
Division: Divides one value by another: ... In the example below, we use the assignment operator (=) to assign the value 10 to a variable called x: Example. int x = 10;
Feb 10, 2024 · The division assignment operator (/=) is an incredibly useful operator in C that allows you to divide a variable by another value and assign the result back to the original variable in one concise statement. However, there are some intricacies to using /= properly and effectively that are important to understand.
Division Assignment Operator In C (/=) The division assignment operator (/=) first divides the current/ initial value of the variable mentioned on its left side by the value given on its right. Then, the revised value/ result is assigned back to the variable on the left.
In this C++ tutorial, you shall learn about Division Assignment operator, its syntax, and how to use this operator, with examples. C++ Division Assignment. In C++, Division Assignment Operator is used to find the division of the variable (left operand) by a value (right operand) and assign the resulting quotient to this variable (left operand).
Arithmetic operators are used to perform common operations like addition, subtraction, multiplication, division, etc. The following table specifies symbol, example, and description for each of the Assignment Operator in C programming.
Jul 2, 2024 · Assignment Operators in C are used to assign values to the variables. The left side operand is called a variable and the right side operand is the value. The value on the right side of the "=" is assigned to the variable on the left side of "=". In this C tutorial, we'll understand the types of C programming assignment operators with examples.
C /= A is equivalent to C = C / A %= Modulus AND assignment operator. It takes modulus using two operands and assigns the result to the left operand. C %= A is equivalent to C = C % A <<= Left shift AND assignment operator. C <<= 2 is same as C = C << 2 >>= Right shift AND assignment operator. C >>= 2 is same as C = C >> 2 &= Bitwise AND ...
Assignment. Assignment. Statements. Expression statement Block statement. ... C - Division: / Division is a matematical operation, it can be used for integers and ...
Certainly! In C programming, assignment operators are used to assign values to variables. Here are some common assignment operators in C along with examples and outputs: 1. Simple Assignment (=): - The basic assignment operator assigns the value on the right-hand side to the variable on the left-hand side. c #include <stdio.h> int main() {int x ...